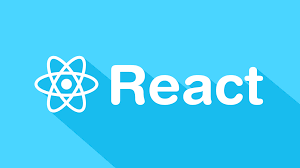
import styled from "styled-components";
//전역 스타일 설정
const GlobalStyle = createGlobalStyle`
button{
padding:5px;
}
//Styled Components로 컴포넌트를 만들고
const BlueButton = styled.button`
background-color: blue;
color: white;
`;
//만들어진 컴포넌트를 재활용해 컴포넌트를 만들 수 있다
const BigBlueButton = styled(BlueButton)`
padding: 10px;
margin-top: 10px;
//마우스를 올려놨을때
&:hover{
background-color: skyblue;
color:blue;
}
`;
//Props로 조건부 렌더링하기
const Button3 = styled.button`
background-color: ${(props) => (props.red ? "red" : "black")};
`;
export default function App() {
// React 컴포넌트를 사용하듯이 사용
return (
<>
<GlobalStyle>
<BlueButton>Blue Button</BlueButton>;
<br />
<BigBlueButton>Big Blue Button</BigBlueButton>
<br />
<Button3 red> button</Button3>
</>
}